WordPress REST API Features and Functions
Image
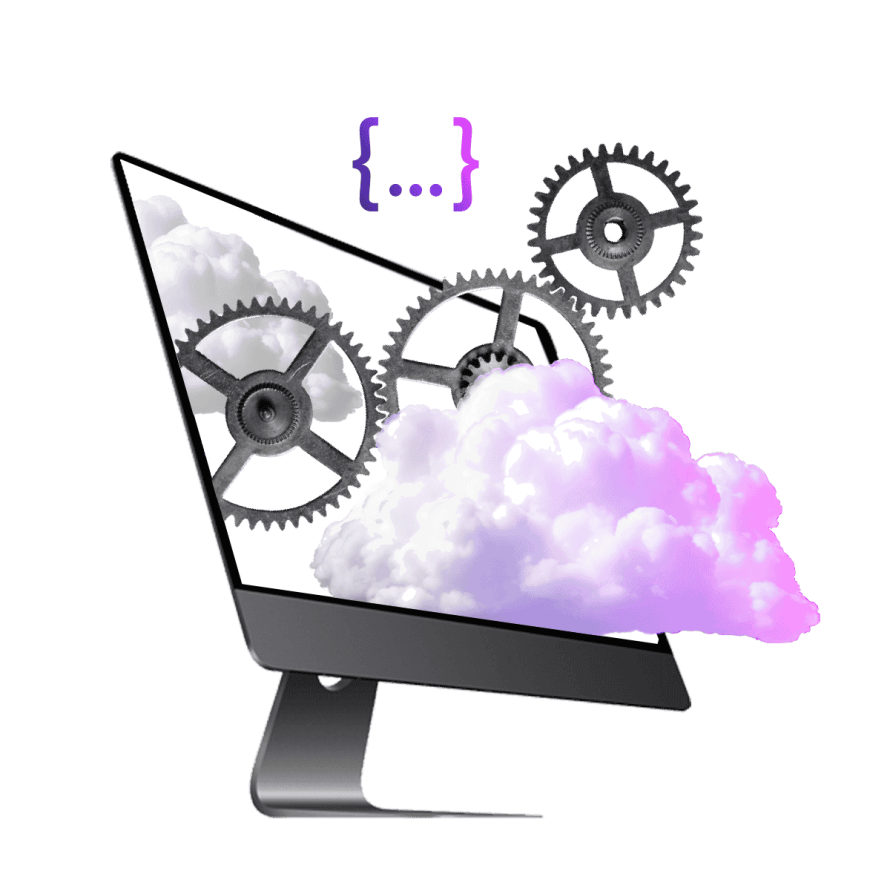
Dynamic and engaging user experiences are the bread and butter of customer satisfaction and business success. If you're a WordPress developer eager to elevate your projects, mastering the WordPress REST API is an amazing tool for that.
This powerful interface allows you to interact with WordPress from outside the traditional confines of themes and plugins, opening up a world of custom development possibilities. From building sophisticated web applications to integrating with external services, the WordPress REST API is your gateway to innovation.
TL;DR: The WordPress REST API turns your WordPress site into a data source that any app can tap into. This means you can build modern apps that use WordPress content, connect WordPress to other tools or create custom mobile apps – all while keeping WordPress's easy-to-use admin interface for content management.
What Is the WordPress REST API?
First of all, an API (application programming interface) basically means a set of rules that lets programs “talk” to each other and exchange data. And so, the WordPress REST API allows other websites, applications or plugins to interact with your WordPress data. The REST (representational state transfer) part is a fancy way of saying there’s a standard set of HTTP methods that let you do things like:
- GET to retrieve or “get” information, i.e. getting all posts.
- POST to create new data, i.e. creating a new post.
- PUT (or PATCH) to update existing data, i.e. editing a post.
- DELETE to remove data, i.e. deleting a post.
This interaction happens over the web, using URLs (just like how you visit a webpage), but instead of returning an HTML webpage, WordPress returns data in a format called JSON (JavaScript object notation). JSON is just a simpler, text-based way to structure data so machines (and humans, if you look closely) can read it.
With the WordPress REST API, developers can:
- Build "headless" WordPress websites – use WordPress as a backend while building the front end with React, Vue.js, Angular, or other modern frameworks. This makes sites faster and more dynamic.
- Develop mobile apps – instead of creating content in a separate system, mobile applications can fetch WordPress posts, pages and user data in real time via API requests.
- Integrate with third-party services – connect WordPress to platforms like CRM systems, social media, e-commerce stores or any other web service that supports APIs.
- Automate content management – schedule posts, fetch analytics or update content without manual intervention by running automated scripts.
The Core Concepts of The WordPress REST API
When you use a REST API, you typically make requests to certain endpoints, which is just a URL that represents a specific piece of data or resource in your WordPress site:
- Almost all REST API endpoints start with https://yoursite.com/wp-json/ (the root of the API).
- The next part is often wp/v2/, indicating the version of the API (referred to as the route).
- After that, you specify what you want, like posts, pages, categories, etc.
So, to get a list of all your posts, you might go to:
https://yoursite.com/wp-json/wp/v2/posts
You can test this in your browser or use tools like Postman or cURL for more complex requests.
Setting Up and Authenticating REST API Requests
While public data (such as publicly published posts, pages and media) can be fetched without authentication, creating, updating or deleting content requires you to authenticate your requests. WordPress supports several authentication methods:
- Cookie-based authentication: Ideal for requests made from the WordPress admin area, like AJAX calls in themes or plugins. It uses the existing logged-in user session but isn't suitable for external applications.
- JWT (JSON Web Tokens): Offers stateless authentication and is particularly useful for decoupled applications. Tokens include user information and are signed to prevent tampering.
- Basic authentication: Involves sending the username and password with each API request. While simple, it's not recommended for production due to security risks, especially if not using HTTPS.
- OAuth authentication: A more secure method that allows users to authorize applications without sharing passwords. However, it's more complex to implement and often overkill for small projects.
- Application passwords: Introduced in WordPress 5.6, this method lets you generate unique passwords for applications. It's a good balance between security and ease of use.
Please note: Some security plugins or server settings might block API requests. Check logs and configurations if problems persist.
Extending the API: Custom Endpoints and Post Types
One of the most powerful aspects of the WordPress REST API is its extensibility. By creating custom endpoints and post types, you can tailor the API to meet the specific needs of your application, providing more flexibility and control over the data you interact with.
Custom post types allow you to organize and manage content beyond the default posts and pages. For instance, if you're developing a portfolio site, you might create a “Projects” post type. Here's a very basic snippet of how you can register a custom post type:
function create_project_post_type() {
register_post_type('project',
array(
'labels' => array(
'name' => __('Projects'),
'singular_name' => __('Project'),
),
'public' => true,
'has_archive' => true,
'show_in_rest' => true, // Enables REST API support
'supports' => array('title', 'editor', 'thumbnail'),
)
);
}
add_action('init', 'create_project_post_type');
By setting 'show_in_rest' => true, you make your custom post type accessible via the REST API, allowing you to perform CRUD operations on your "Projects" just like standard posts.
Sometimes, the default REST API endpoints don't cover all the functionality you need. In such cases, you can create custom endpoints. This is especially useful for complex operations or aggregating data from multiple sources.
Here's a simple example of registering a custom endpoint:
function register_custom_endpoints() {
register_rest_route('custom/v1', '/featured-projects', array(
'methods' => 'GET',
'callback' => 'get_featured_projects',
));
}
add_action('rest_api_init', 'register_custom_endpoints');
function get_featured_projects($request) {
$projects = get_posts(array(
'post_type' => 'project',
'meta_query' => array(
array(
'key' => 'is_featured',
'value' => true, // Treat as boolean
'compare' => '='
)
)
));
return rest_ensure_response($projects);
}
This code creates a new GET endpoint at /wp-json/custom/v1/featured-projects that returns a list of featured projects. Defining this custom namespace in the WordPress REST API (instead of just using wp/v2) helps avoid conflicts, organize API endpoints, enable versioning, improve security and optimize performance. It’s considered best practice when extending the WordPress REST API with custom functionality.
Optimizing REST API Performance with Pantheon's WebOps Platform
For fast and reliable WordPress applications, performance is crucial, particularly when you're using the REST API for dynamic content delivery. Pantheon offers a suite of tools and features designed to optimize your REST API performance, ensuring that your custom developments run smoothly and efficiently.
Scalable Infrastructure
Pantheon's infrastructure is built on a container-based system that automatically scales to handle traffic spikes. This means your REST API endpoints remain responsive even under heavy load. With Pantheon's multizone high-availability architecture, your API requests are distributed across multiple data centers, reducing latency and improving response times globally.
Content Delivery Network (CDN) Integration and Advanced Caching Mechanisms
One of the key aspects of API performance is how well you manage caching. Pantheon provides advanced caching strategies at the edge using its Global CDN. To further enhance backend performance, we recommend Object Cache Pro, which is available to all Performance and Elite sites on Pantheon. It optimizes WordPress database queries by storing frequently accessed data in memory, significantly reducing query load and improving response times.
By leveraging this high-performance Redis-based object caching solution, you can ensure faster API responses and a more scalable WordPress backend.
Optimized Development Workflow
Pantheon streamlines your development workflow with tools like Dev, Test and Live environments.
Brandfolder Image
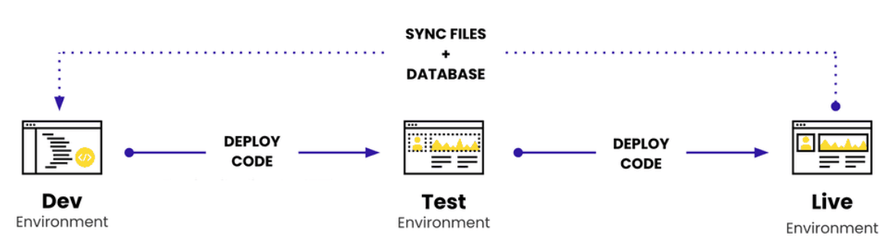
You can develop and test your REST API integrations in isolated environments without affecting your live site. This separation ensures that performance optimizations and updates can be thoroughly vetted before deployment, minimizing risks and downtime.
Automated Performance Monitoring
With Pantheon, you gain access to real-time performance analytics. Tools like New Relic APM are integrated into the platform, allowing you to monitor your REST API's performance metrics. You can identify bottlenecks, slow queries or inefficient code paths that may be impacting your API's responsiveness. Armed with this data, you can make informed optimizations to enhance performance.
Image
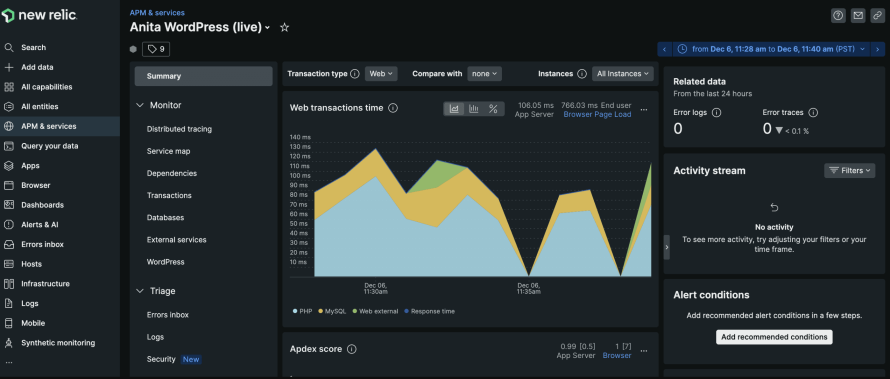
Secure and Efficient Routing
Pantheon ensures that all requests, including your REST API ones, are securely routed through HTTPS with HTTP/2 support.
Image
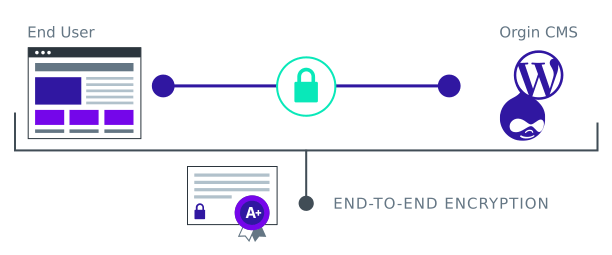
This modern protocol enhances performance by allowing multiple simultaneous requests over a single connection, reducing overhead and improving load times for API calls. The platform also automates the certificate provisioning and renewal process, eliminating the need for manual certificate management.
Implementing Strong Security Measures for Your REST API
As the API exposes endpoints that can manipulate site data, implementing strong security measures ensures that only authenticated and authorized users can interact with your site.
The first step is to ensure all API communications occur over HTTPS. By encrypting data transmission, HTTPS prevents man-in-the-middle attacks and eavesdropping. Obtain an SSL certificate from a trusted provider or use services like Let's Encrypt. This encryption safeguards sensitive information like authentication tokens and user data.
If you’re using Pantheon, you don’t have to worry about that. Pantheon offers fully managed and free HTTPS, ensuring secure website connections.
Image
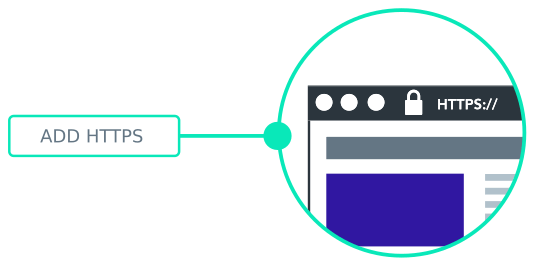
Make sure to protect against common vulnerabilities by:
- Sanitizing incoming data before storing it in the database. Use functions like sanitize_text_field() or sanitize_email() to clean user input and prevent malicious data from being saved.
- Escaping output before displaying or returning it. Use esc_html(), esc_attr(), or esc_url() to ensure data is safely rendered in HTML, API responses, or JavaScript.
This prevents SQL injection, cross-site scripting (XSS) and other injection attacks.
Also, use nonces (numbers used once) to verify that the request comes from a legitimate source. This protects against CSRF (cross-site request forgery) attacks.
If certain REST API endpoints aren't needed, disable them to reduce the attack surface. You can use plugins like Disable REST API. Additionally, remove or obscure sensitive data from API responses. Filter the data returned (like user_email, roles, caps, meta_key, meta_value, etc.) to only include what's necessary.
Try implementing rate limiting and throttling to restrict the number of requests a user or IP can make within a given timeframe. This helps in preventing API abuse.
Here’s how you can implement it:
- Server-level configuration: If you’re using Nginx, you can configure rate limiting in your nginx.conf file:
limit_req_zone $binary_remote_addr zone=api_limit:10m rate=10r/s;
server {
location /wp-json/ {
limit_req zone=api_limit burst=20 nodelay;
}
}
This limits each IP to 10 requests per second, with a burst allowance of 20. For Apache, use mod_ratelimit or mod_security to control request rates.
- Security plugins (easy option): If you’re using WordPress, plugins like Limit Login Attempts Reloaded can provide API rate limiting without requiring server configuration.
Also, look into enhancing security with HTTP headers. Security headers help defend against attacks like cross-site scripting (XSS) and man-in-the-middle attacks. Here’s how to implement them:
- Content security policy (CSP): CSP restricts what content (e.g., JavaScript, images, fonts) can load on your site, preventing malicious code injection. This protects against XSS). Example for Nginx:
add_header Content-Security-Policy "default-src 'self'; script-src 'self' 'https://trustedscripts.com'; object-src 'none';";
This ensures only scripts from your domain and trustedscripts.com are allowed, blocking unauthorized third-party scripts.
- Strict-transport-security (HSTS): HSTS ensures all connections use HTTPS, preventing downgrade attacks. Example for Apache:
Header always set Strict-Transport-Security "max-age=31536000; includeSubDomains; preload"
This tells browsers to enforce HTTPS for one year across all subdomains.
Accelerate your REST API development with Pantheon
Mastering the WordPress REST API allows you to create dynamic, customized web experiences that stand out. With this powerful tool, you can build modern applications, integrate with external services and offer exceptional functionality to your users.
Don't forget that Pantheon enhances this journey by providing a powerful, high-performance environment tailored for WordPress development. With features that optimize performance and security, Pantheon enables you to focus on innovation without worrying about infrastructure hurdles.
Try Pantheon today and explore how it can accelerate your API development and help you deliver exceptional results!