WP_Query Hacks for Lightning-Fast WordPress Sites
Image
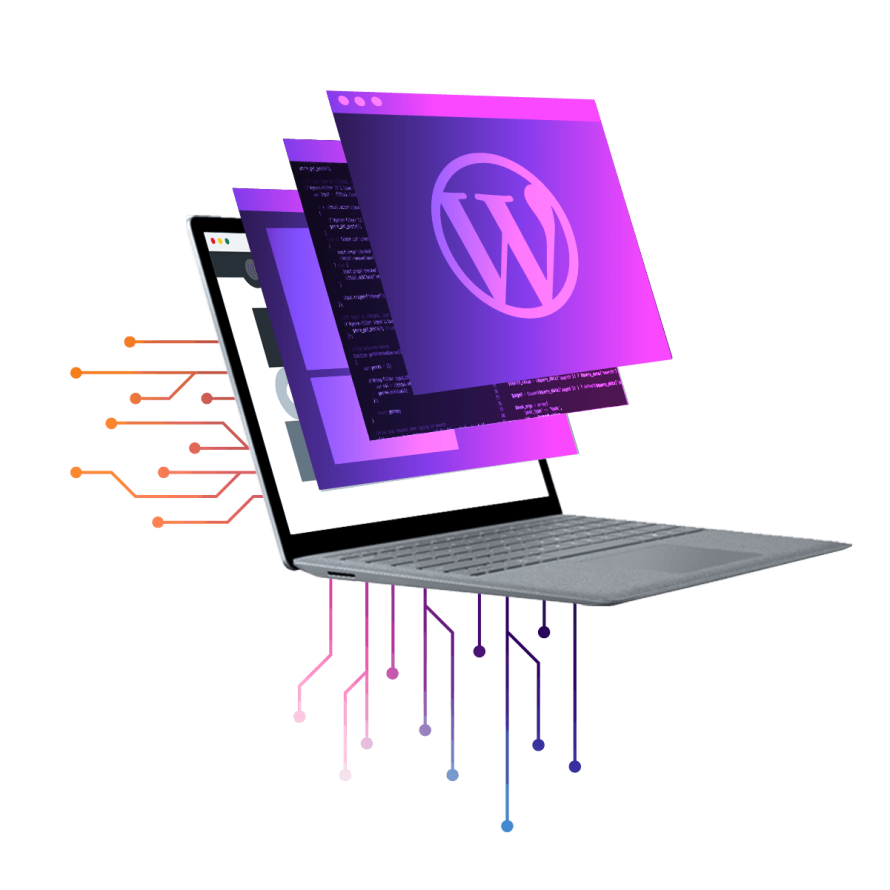
For WordPress developers and site owners, mastering WP_Query can unlock significant performance gains. WP_Query is the powerhouse behind WordPress's content retrieval system. It allows fetching posts, pages and custom content types with remarkable flexibility. However, inefficient queries can bog down your site, leading to sluggish performance and frustrated users.
In this article, we’ll take a look at advanced WP_Query techniques that enhance precision and boost performance. Plus, we'll share expert tips on debugging and fine-tuning complex queries to ensure your site runs at peak efficiency!
What Is WP_Query and How Do You Use It?
WP_Query is essentially a PHP class that allows you to craft custom queries to the WordPress database. It gives you the flexibility to fetch exactly the content you need based on parameters like post types, categories, tags, custom fields and more. Whether you're looking to display a list of upcoming events, showcase products from a specific category or highlight blog posts by a particular author, WP_Query makes it possible.
Using WP_Query is straightforward yet incredibly powerful. Here's a simple example:
$args = array(
'post_type' => 'post',
'posts_per_page' => 5,
'category_name' => 'technology',
);
$query = new WP_Query( $args );
if ( $query->have_posts() ) {
while ( $query->have_posts() ) {
$query->the_post();
// Your code to display the post goes here
}
wp_reset_postdata();
} else {
// No posts found
}
In this snippet, we're querying the database for the latest five posts in the "technology" category. The $args array defines the parameters of our query and WP_Query processes these parameters to fetch the desired posts.
To use WP_Query effectively, you need to:
- Start by deciding what content you need. WP_Query supports a wide range of parameters, allowing you to filter posts by date, custom fields, taxonomies and more.
- Create a new instance of WP_Query with your parameters.
- Use a loop to go through the posts returned by your query.
- Always reset the post data to ensure other parts of your page function correctly after your custom loop,
Effective use of WP_Query can significantly reduce database load and improve page load times. As we take a closer look into advanced WP_Query arguments in this post, you'll discover techniques to optimize your queries for both precision and speed, ensuring your WordPress site runs lightning-fast.
Advanced WP_Query Arguments for Precision and Performance
Conditional Tags and Parameter Combinations
By combining multiple parameters, you can create queries that are finely tuned to your requirements. For instance, you might want to fetch posts that belong to multiple categories or have certain tags. Here's how you can achieve that:
$args = array(
'category__and' => array(2, 6),
'tag__in' => array('featured', 'popular'),
'posts_per_page' => 10,
);
$query = new WP_Query( $args );
In this example, we're fetching posts that are in both categories 2 and 6 and have either the “featured” or “popular” tag.
Custom Field (Meta) Queries
Meta queries allow you to filter posts based on custom field values. This is incredibly useful for custom post types that have additional metadata. For example:
$args = array(
'post_type' => 'product',
'meta_query' => array(
array(
'key' => 'price',
'value' => 50,
'compare' => '>',
'type' => 'NUMERIC',
),
),
);
$query = new WP_Query( $args );
Here, we're retrieving products with a price greater than 50. Using the 'type' => 'NUMERIC' argument ensures that the comparison is done numerically, not alphabetically.
Date Queries for Time-Based Filtering
Need to fetch posts from a specific time period? Date queries are your friend:
$args = array(
'date_query' => array(
array(
'after' => 'January 1st, 2024',
'before' => 'December 31st, 2024',
'inclusive' => true,
),
),
'posts_per_page' => -1,
);
$query = new WP_Query( $args );
This query fetches all posts published in the year 2024.
Enhancing Performance with Caching
While crafting advanced queries, it's essential to consider performance. One way to optimize is by leveraging WordPress's built-in caching mechanisms:
$args = array(
'post_type' => 'event',
'posts_per_page' => 20,
'update_post_meta_cache' => false,
'update_post_term_cache' => false,
);
$query = new WP_Query( $args );
By setting 'update_post_meta_cache' and 'update_post_term_cache' to false, you reduce the number of database queries, which can speed up your site, especially when meta or term data isn't needed.
Utilizing Pagination Parameters
For content-heavy sites, efficient pagination is essential:
$paged = ( get_query_var('paged') ) ? get_query_var('paged') : 1;
$args = array(
'post_type' => 'post',
'posts_per_page' => 10,
'paged' => $paged,
);
$query = new WP_Query( $args );
Proper pagination improves user experience and reduces server load by limiting the amount of data processed at once.
Mastering Meta Queries and Custom Taxonomy Filters
Meta queries let you filter posts based on custom field values stored in the post meta table. This is incredibly useful when working with custom post types that have additional metadata. For example, suppose you have a custom post type called event with a custom field event_date. You can query upcoming events like this:
$args = array(
'post_type' => 'event',
'meta_query' => array(
array(
'key' => 'event_date',
'value' => date('Y-m-d'),
'compare' => '>=',
'type' => 'DATE',
),
),
'orderby' => 'meta_value',
'order' => 'ASC',
);
$query = new WP_Query( $args );
In this example, we're fetching events with an "event_date" on or after today ordering them chronologically.
Meanwhile, custom taxonomies allow you to create bespoke classification systems beyond the default categories and tags. Using WP_Query, you can filter posts based on these custom taxonomies. For instance, if you have a custom taxonomy "genre" for a "book" post type, you can retrieve all science fiction books like so:
$args = array(
'post_type' => 'book',
'tax_query' => array(
array(
'taxonomy' => 'genre',
'field' => 'slug',
'terms' => 'science-fiction',
),
),
);
$query = new WP_Query( $args );
Here, we're querying all books that have been assigned the "science-fiction" genre.
For even more precise results, you can combine meta queries with taxonomy filters. Suppose you want to find products that are on sale (a custom field) in specific categories (a taxonomy). Here's how:
$args = array(
'post_type' => 'product',
'meta_query' => array(
array(
'key' => 'on_sale',
'value' => '1',
'compare' => '=',
),
),
'tax_query' => array(
array(
'taxonomy' => 'product_cat',
'field' => 'slug',
'terms' => array( 'electronics', 'appliances' ),
'operator' => 'IN',
),
),
);
$query = new WP_Query( $args );
This query fetches all products marked as “on sale” that belong to either the “electronics” or “appliances” categories.
Here are some extra tips for optimal performance:
- Index your meta keys: Frequently queried custom fields should be indexed in your database to speed up meta queries.
- Use relation parameters: In both meta and tax queries, you can use the 'relation' parameter ('AND' or 'OR') to combine multiple conditions efficiently.
- Limit result sets: Always specify 'posts_per_page' to limit the number of results and reduce server load.
Optimizing WP_Query for Scale: Techniques for High-Traffic Sites
When your WordPress site starts attracting a substantial amount of traffic, the efficiency of your database queries becomes critically important. Poorly optimized queries can lead to increased server load, slower response times and a degraded user experience. Thankfully, there are several techniques you can employ to optimize WP_Query for high-traffic scenarios, ensuring your site remains lightning-fast even under heavy load.
Select Only What You Need
By default, WP_Query retrieves all fields from the database. However, in many cases, you don't need all this information. You can optimize your queries by specifying only the fields you require.
Use Cached Queries
By storing the results of expensive queries, you can serve data quickly without hitting the database every time. WordPress has built-in caching mechanisms, but you can enhance this with transient APIs or external caching plugins:
$cached_posts = get_transient( 'my_custom_query' );
if ( false === $cached_posts ) {
$args = array(
'post_type' => 'product',
'posts_per_page' => 50,
);
$query = new WP_Query( $args );
$cached_posts = $query->posts;
set_transient( 'my_custom_query', $cached_posts, HOUR_IN_SECONDS );
}
This code checks for a cached version of the query results and only runs a new query if none is found, significantly reducing the database load.
Optimize Database Indexes
For sites with large amounts of data, database optimization is crucial. Ensure that your custom fields (meta keys) and taxonomies that are frequently queried are properly indexed. While WordPress indexes some fields by default, custom fields often require manual indexing.
Limit Query Scope
Restrict your queries to search only what's necessary. For example, if you only need published posts, specify that:
$args = array(
'post_status' => 'publish',
'posts_per_page' => 10,
);
$query = new WP_Query( $args );
By limiting the scope, you reduce the amount of data the database needs to search through.
Offload Intensive Queries
Consider offloading heavy queries to asynchronous processes or background tasks. Tools like WP-Cron or external services can handle data processing without impacting the user experience.
Avoid N+1 Query Problems
Be cautious of loops within loops, which can lead to a large number of database queries. For example, calling get_post_meta() inside a loop can be inefficient. Instead, retrieve all necessary metadata in a single query:
Utilize Object Caching
If you're on a hosting platform that supports object caching (like Redis or Memcached), ensure it's enabled and properly configured. Object caching stores the results of database queries in memory, allowing for faster retrieval.
Leverage Pantheon's Optimized Infrastructure
Hosting providers like Pantheon offer optimized environments specifically designed for high-traffic WordPress sites. Their infrastructure includes advanced caching layers, scalable resources and New Relic performance monitoring to keep your site running smoothly.
Debugging and Fine-Tuning Complex Queries for Peak Performance
Even the most carefully constructed WP_Query instances can sometimes lead to unexpected results or slow performance. Debugging and fine-tuning these complex queries is essential to ensure your WordPress site runs at peak efficiency. Here are some expert strategies to help you identify and resolve issues with your queries:
- Enable debugging tools: Start by activating WordPress's built-in debugging features. In your wp-config.php file, add the following lines:
define( 'WP_DEBUG', true );
define( 'SAVEQUERIES', true );
With SAVEQUERIES enabled, WordPress stores all database queries in the $wpdb->queries array. This allows you to review each query and its execution time and identify any unnecessarily queries taxing your database.
- Use query monitoring plugins: Plugins like Query Monitor provide a user-friendly interface to inspect and analyze your database queries. They highlight slow or duplicate queries, helping you pinpoint inefficiencies quickly.
- Use caching mechanisms to store query results: Use a persistent object cache like Redis or Memcached to reduce database load. Pantheon offers support for Redis that you can use for persistent object caching in WordPress. This feature is particularly beneficial for high-traffic sites where database efficiency is crucial.
- Profile query execution time: By profiling your queries, you can pinpoint which ones are consuming the most time and resources and then take steps to optimize them for better performance. You can measure the execution time of a query by capturing the start and end times using the microtime(true) function in PHP. If the execution time is significantly high (e.g., over 0.5 seconds), it indicates that the query may be inefficient and could benefit from optimization.
- Test queries directly: Use tools like phpMyAdmin or WP-CLI to run and examine your queries directly against the database. This helps you understand the raw SQL behind WP_Query and identify any inefficiencies.
Elevate Your WordPress Projects With Pantheon’s Optimized Infrastructure
Mastering WP_Query is important for optimizing a WordPress site. By implementing advanced arguments, using meta queries and fine-tuning complex queries, you can significantly enhance your site's speed and performance. These optimizations improve the user experience and give you a competitive edge in search engine rankings.
However, even the most optimized queries need a powerful hosting environment so you can really experience the difference. With a platform designed specifically for high-performance WordPress sites, Pantheon ensures that your hard work in optimizing WP_Query translates into a lightning-fast, reliable experience for your users.
As Marc Cracco, CTO at Wondersauce, highlights:
“Working with Pantheon has been crucial. We don’t want a dedicated DevOps team on the Golf.com project from a cost and staffing perspective. So having Pantheon fill that role and being able to support all the goals and initiatives when needed is very crucial.”
Get started with Pantheon today to amplify your site's performance now!