Understanding and Implementing Website Security: Application Layer
Image
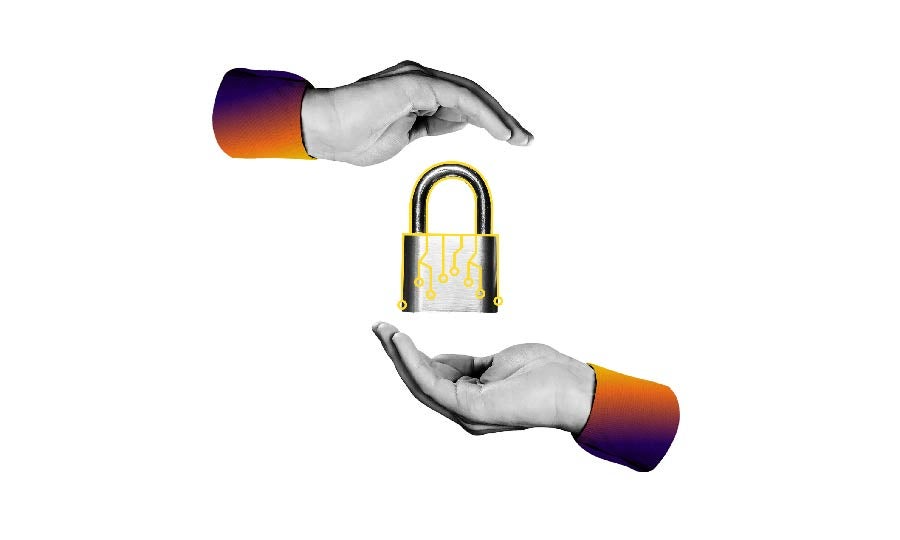
We're going to turn our focus to the application layer and look at the kinds of threats we need to guard against in Drupal and WordPress.
Enter Panama
Interestingly enough, the wider world has also been hearing about security practices related to Drupal and WordPress; the Panama Papers leak was quite plausibly caused by out-of-date, insecure versions of WordPress and Drupal. The hack of the Panamanian law firm, Mossack Fonseca, yielded over 11 million documents and 2.6 terabytes of data that has incriminated corrupt leaders the world over. Mossack Fonseca maintained two websites with huge security flaws that could have led to the breach; a highly vulnerable WordPress site and a highly vulnerable Drupal site. Both of those vulnerabilities should have been prevented by proper application layer coding and could have also been mitigated by organizational best practices like keeping up with security updates, which weâll cover in Part 7 of this series.
Understanding the Threats
There are a number of common vulnerability patterns for all web applications. The Open Web Application Security Project (OWASP) maintains a Top 10 List of the most critical web application security flaws. Itâs referenced by many standards, books, tools, and organizations, including MITRE, PCI DSS, DISA, FTC, and many more. The list was first established in 2003 and has been updated periodically since then. The current list was established in 2013 and lists the following as the 10 most serious security flaws for web applications:
Security Benefits of Drupal and WordPress
One of the many benefits of using a well-established CMS like WordPress or Drupal is that we gain from the security knowledge, best practices and vigilance of these communities and their respective security teams. Additionally, the built-in user management, permissions and session management in both CMSs greatly mitigates a number of these vulnerabilities. Proper configuration and use of the APIs handle the rest. In Parts 5 and 6, Securing Drupal and Securing WordPress, weâll cover those practices in more specificity. In this post, weâll start by looking at three of the most dangerous things we all need to guard against in our own code: Injection, XSS and CSRF.
Digital Literacy
Even if you arenât directly involved in writing code for WordPress or Drupal, understanding these patterns is an important part of digital iteracy. Knowing the basics of how these attacks work will make you a better educated website owner, administrator and user.
Understanding Then Action
If you write code for Drupal or WordPress, itâs your duty to learn how to prevent all of these attacks. If you administer a site, educate yourself on how to pick secure modules and plugins and establish a procedure for making security updates. Upcoming posts Part 5: Securing Drupal, Part 6: Securing WordPress and Part 7: Organizational Security will give you the tools to do so.
SQL Injection
Injection has been one of the top threats in every edition of the OWASP 10 since it first came out. XKCD has a classic comic that does an excellent job summarizing SQL Injection.
In this theoretical example, her sonâs first name is
Robertâ); DROP TABLE Students; --
An unusual name, to be sure. Anyone typing this information into the âFirst Nameâ field of the schoolâs administrative system could cause a major problem. The code to store this information in the database behind the form might look something like this:
INSERT INTO table_name (column1,column2,column3,...) VALUES (âvalue1â,âvalue2â,âvalue3â,...);
Plugging little Bobbyâs name into that code turns the statement into:
INSERT INTO Students (first_name, last_name, ...) VALUES (âRobertâ); DROP TABLE Students; --â, âLast Nameâ, ...);
On running the above command, the database would:
Insert a record with the first name of âRobertâ:
INSERT⊠VALUES (âRobertâ)
Complete that statement and be ready for the next command:
â);
Run the next statement:
DROP TABLE Students;
Ignore everything afterwards to prevent any errors:
--
The end effect? All student data is wiped out.
Never Trust User Input
The lesson from SQL Injection is to never trust user input. Instead of running that SQL statement directly, the system should have first examined it for any potential malicious or error-causing input and guarded against accordingly. Both WordPress and Drupal have such a system, but that doesnât mean that every module, plugin or theme author has used that system properly.
Cross Site Scripting (XSS)
Cross Site Scripting is the next serious vulnerability that all Drupal and WordPress professionals need to understand. Most modern websites use JavaScript on every page load for transitions, animations, modal dialogues, dynamic forms and more. XSS happens when a user visits a page that contains malicious JavaScript that will run commands on their behalf. If that user happens to be logged into the site, the JavaScript could do any action the logged-in user is able to do. If youâve ever seen a security demonstration that ends with something like alert('xss') and wondered what thatâs about, the point is that anything you can do in the browser could have just happened; adding users, inserting content, uploading files, etc. As Greg Knaddison of the Drupal Security Team famously put it: âAnything you can do XSS can do betterâ.
Consider the following code snippet on a search.php page:
<?php echo "You searched for: " . $_GET["search_terms"]; ?>
Visiting that page with this URL:
http://example.com/search.php?search_terms=<script>Bad Code Here</script>
would run the bad code.
While this can be malicious in and of itself, XSSâs power is magnified by the fact that Drupal and WordPress are managed by website visitors. If the code is crafted with that in mind (and it will be), exceedingly bad things can happen. Of course, most people visiting a page infected by XSS wonât be site administrators, but once a privileged user visits the page, the attack will runâ and absolutely anything could happen. Uploading malicious files that can be used to control the server and/or infect other site visitors? Certainly. Subtly and silently creating a new user with full admin privileges that a hacker can use later at their leisure? Absolutely.
Escaping Output
Once again, XSS reminds us that user input cannot be trusted. Preventing XSS also requires us to properly encode and escape everything that is written out to the browser. Both WordPress and Drupal include APIs to sanitize and prevent XSS, but those tools have not been used properly by every single person writing code for Drupal and WordPress.
Cross Site Request Forgery (CSRF)
Whereas XSS attacks the current site, CSRF uses code on one site to launch an attack against another site. As WordPress and Drupal professionals, we have a dual responsibility to protect our sites from being used to launch these attacks as well as to make sure theyâre not vulnerable to being attacked.
Consider the following simple example:
<a href="https://bank.com/transfer.do?acct_number=1234567890&amount=10000">View my pictures!</a>
Anyone seeing this page would see a link to âView my picturesâ. Clicking that link would take them to another URL: https://bank.com/transfer.do?acct_number=1234567890&amount=10000
. If that user happened to be a customer of Bank.com and Bank.com was vulnerable to this kind of attack, $10,000 would be transfered from their account to account number 1234567890.
Once again, this requires a few circumstances to be true and most users will not lose $10,000 from their bank account by visiting this page. However, getting this to succeed just once would turn into a $10,000 payout for the attacker. If this attack works once in every 10,000 times, an attacker who gets this code viewed 100,000 or 1,000,000 times could make a LOT of money. This means an attacker who can find such a vulnerability is highly motivated to expose it to as many people as possible, which reminds us that every website is a target.
Verifying Origin and Intent
Both WordPress and Drupal are written in ways to prevent being a target of CSRF, but once again, correct techniques must be used with any custom code. Proper use of WordPress nonces and Drupalâs Form API and tokens are necessary to prevent either from being used to launch CSRF attacks. Both of these systems add bits of unique data to requests in order to verify that requests come from the site they appear to come from.
Application Layer Threats
With the combined dominance of WordPress and Drupal, all of us have a serious responsibility to make the internet safer. Understanding the OWASP 10 threats and the tools our CMSs have to combat them is an important starting point. Next up, weâll cover specific security recommendations for Drupal, followed by security recommendations for WordPress.