The Pantheon Blog
Brandfolder Image
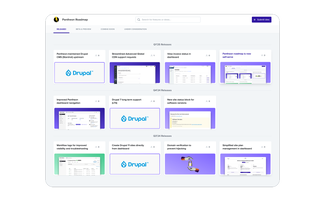
Latest insights
Enhanced Drupal Integration for Content Publisher
Read MoreA WordPresser goes to DrupalCon Atlanta 2025
Read MoreAnnouncing 2025 Pantheon Partner Summit Award Winners
Read MoreA New Era for Customer Experience: Introducing Pantheon’s CCO
Read MoreConnecting Enrollment and Web Teams for Student Success
Read MoreWhat's Cooking at Pantheon's DrupalCon Booth 2025
Read MoreWeb Governance 101: Top Five Lessons from Brown and Harvard
Read MorePantheon’s External Roadmap: Transparency, Collaboration, and the Future of WebOps
Read MoreContent Publisher just got better: meet Preview Editor
Read MoreDrupal CMS: It's About Time
Read MoreWhat can a WordPresser learn from Drupal CMS?
Read MoreScoring with a Seamless CMS Migration: A Healthcare Playbook for Digital Excellence
Read More